需求:表格有一列为勾选框列,表格下面有单独的按钮本页勾选和全部勾选,跨页状态可以保存回显,如下图所示:
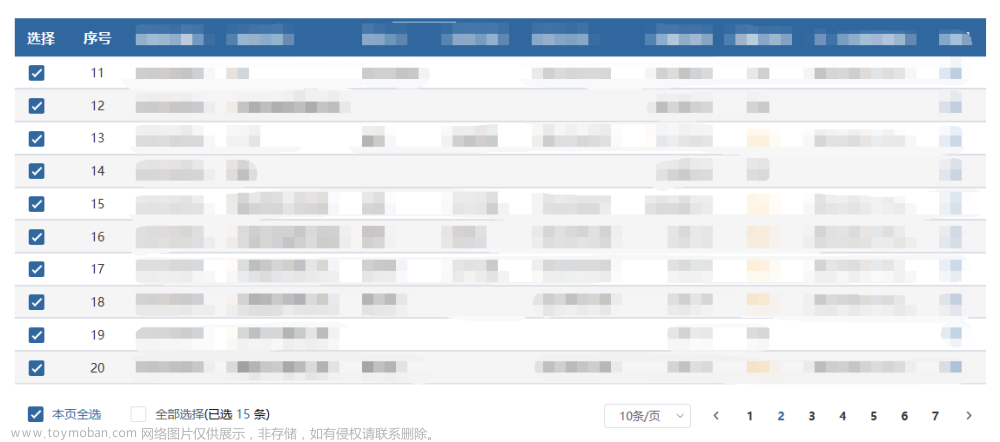
思路:使用一个数组[]存储每一页是否全选的状态,再使用{}来存储数据的所有选中状态,其中key为对应的页码,value为每一页的选中数据【核心❗】
1、el-table表格每一行绑定状态,这里没有使用el-table自带的type为selection的多选框
<el-table-column
label="选择"
width="60"
align="center"
>
<template slot-scope="scope">
<el-checkbox v-model="scope.row.isSelect" :label="scope.row" @change="(val) => handleSelect(val, scope.row)"><br></el-checkbox>
</template>
</el-table-column>
<div style="float: left; line-height: 28px" class="ml15">
<el-checkbox v-model="currentCheck" @change="chooseCurrent">本页全选</el-checkbox>
<el-checkbox v-model="allCheck" @change="chooseAll" >全部选择</el-checkbox>(已选 <span class="txt_primary">{{sum}}</span> 条)
</div>
2、初始化准备好各种数据
data() {
return {
tableData: [], // 表格
allCheckedList:{}, // 所有选中数据
currentChecked:[], // 每页状态
tablePage:[10, 1, 500],
currentCheck:false,
allCheck:false,
pageSum:0,
sum:0,
};
},
methods:{
// 加载表格并初始化各项数据
loadTable() {
axios({
method: "post",
url: "",
data: {
ajax: true,
act: "",
},
}).then((response) => {
let resp = response.data;
if (resp.success) {
this.tableData = resp.list;
this.tablePage = resp.pagging;
this.tableData.forEach(item=>{
this.$set(item, 'isSelect', false)
});
this.currentChecked = [];
if(this.tablePage[2]%this.tablePage[0] !=0 ){
this.pageSum = parseInt(this.tablePage[2]/this.tablePage[0]) + 1 ;
} else {
this.pageSum = parseInt(this.tablePage[2]/this.tablePage[0]);
}
for(let i=0; i<this.pageSum;i++){
this.currentChecked.push('false');
}
for(let i=1; i<=this.pageSum; i++){
this.allCheckedList[i] = [];
}
} else {}
}).catch(error=> {});
},}
3、主要的全选、单选、取消勾选等联动逻辑
methods:{
// 本页全选
chooseCurrent(){
if(this.currentCheck){
this.currentChecked[(this.tablePage[1]-1)] = 'true';
this.tableData.forEach(item=>{
this.$set(item, 'isSelect', true);
});
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
this.allCheckedList[this.tablePage[1]] = deepclone(this.tableData);
}
}
} else {
this.currentChecked[(this.tablePage[1]-1)] = 'false';
this.tableData.forEach(item=>{
this.$set(item, 'isSelect', false);
});
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
this.allCheckedList[this.tablePage[1]]= [];
}
}
}
},
// 全部全选
chooseAll(){
if(this.allCheck){
this.currentChecked = [];
for(let key in this.allCheckedList){
this.allCheckedList[key] = [];
}
if(this.tablePage[2]%this.tablePage[0] !=0 ){
this.pageSum = parseInt(this.tablePage[2]/this.tablePage[0]) + 1 ;
} else {
this.pageSum = parseInt(this.tablePage[2]/this.tablePage[0]);
}
for(let i=0; i<this.pageSum;i++){
this.currentChecked.push('true');
}
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
this.allCheckedList[this.tablePage[1]] = deepclone(this.tableData);
}
}
} else {
this.currentChecked = [];
for(let key in this.allCheckedList){
this.allCheckedList[key] = [];
}
for(let i=0; i<this.pageSum;i++){
this.currentChecked.push('false');
}
}
},
// 单行选择
handleSelect(val,row){
if(val){
for (let key in this.allCheckedList){
if(key == this.tablePage[1]){
let hasObj = false;
this.allCheckedList[this.tablePage[1]].forEach(item =>{
if (item.durrec_id == row.durrec_id) {
hasObj = true;
}
})
if (!hasObj) {
this.allCheckedList[this.tablePage[1]].push(row);
}
}
}
} else {
for ( let key in this.allCheckedList){
if(key == this.tablePage[1]){
for(let i = 0; i < this.allCheckedList[this.tablePage[1]].length;i++){
if(row.durrec_id == this.allCheckedList[this.tablePage[1]][i].durrec_id){
this.allCheckedList[this.tablePage[1]].splice(i,1)
}
}
}
}
}
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
if(this.allCheckedList[this.tablePage[1]].length == this.tableData.length){
this.currentChecked[(this.tablePage[1]-1)] = 'true';
} else {
this.currentChecked[(this.tablePage[1]-1)] = 'false';
}
}
}
},
// 计算已选数据
checkSum(){
this.sum = 0;
let currentSum = [];
for(let i = 0; i<this.currentChecked.length;i++){
if(this.currentChecked[i] == 'true' && (i + 1) != this.pageSum){
currentSum.push(this.tablePage[0]);
} else if(this.currentChecked[i] == 'true' && (i + 1) == this.pageSum){
currentSum.push(this.tablePage[2] - this.tablePage[0] * (this.pageSum -1));
} else {
currentSum.push(this.allCheckedList[i+1].length);
}
}
for(let i = 0; i< currentSum.length;i++){
this.sum += currentSum[i];
}
},
},
4、因为只能拿到当前分页的数据,无法拿到所有数据,使用watch监听了一下
watch:{
tableData:{
handler (value) {
// 表格分页后的数据状态
if(this.currentChecked[(this.tablePage[1]-1)] == 'true'){
value.forEach(item=>{
this.$set(item, 'isSelect', true);
});
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
this.allCheckedList[this.tablePage[1]] = deepclone(value);
}
}
this.currentCheck = true;
} else {
for(let key in this.allCheckedList){
if(key == this.tablePage[1]){
if(this.allCheckedList[this.tablePage[1]].length !==0){
this.allCheckedList[this.tablePage[1]].forEach(item =>{
value.forEach(i=>{
if(item.durrec_id == i.durrec_id){
this.$set(i, 'isSelect', true);
}
})
})
} else {
value.forEach(item=>{
this.$set(item, 'isSelect', false);
})
}
}
}
this.currentCheck = false;
}
// 判断全选按钮
let allCheck = true;
this.currentChecked.forEach(checkFlag=>{
allCheck = allCheck && checkFlag == 'true'
})
this.allCheck = allCheck;
// 计算已选数量
this.checkSum();
},
deep:true
},
currentChecked:{
handler(value){
if(value[(this.tablePage[1])-1] == 'true'){
this.tableData.forEach(item=>{
this.$set(item, 'isSelect', true);
})
} else {
this.tableData.forEach(item=>{
this.$set(item, 'isSelect', false);
})
}
this.checkSum();
},
deep:true
},
},
5、回传所有的已选数据,利用分页的请求又拿了一遍数据文章来源:https://www.toymoban.com/news/detail-504743.html
// 批量提醒
allWarning(){
if(this.allCheck){
axios({
method: "post",
url: "",
data: {
ajax: true,
act: "",
check:'T',
},
}).then((response) => {
let resp = response.data;
if (resp.success) {
// ...
} else {
this.$message.error(resp.message);
}
}).catch(error=> {
});
} else {
let choosedIdT = [];
let choosedIdF = [];
for(let i=0; i<this.currentChecked.length; i++){
if(this.currentChecked[i] == 'true'){
axios({
method: 'post', url: '',
data: {
ajax: true, act: '', page: i+1,
}
}).then( response => {
let resp = response.data;
if(resp.success){
const pageList = deepclone(resp.list);
pageList.forEach(item =>{
choosedIdT.push(item.durrec_id);
})
}else{
Valert({msg:resp.message})
}
}).catch(error=> { });
} else {
this.allCheckedList[i+1].forEach(i=>{
choosedIdF.push(i.durrec_id);
})
}
}
setTimeout(()=>{
const ids = choosedIdT.concat(choosedIdF);
axios({
method: "post",
url: "/",
data: {
ajax: true,
act: "",
check:'F',
id:ids.join(","),
},
loading:{ target:".table"}
}).then((response) => {
let resp = response.data;
if (resp.success) {
// ...
} else {
this.$message.error(resp.message);
}
}).catch(error=> { });
},300)
}
},
记录一些繁琐精巧的笨方法😉文章来源地址https://www.toymoban.com/news/detail-504743.html
到了这里,关于【element-ui】使用el-checkbox完成el-table表格数据的全选操作的文章就介绍完了。如果您还想了解更多内容,请在右上角搜索TOY模板网以前的文章或继续浏览下面的相关文章,希望大家以后多多支持TOY模板网!