生成二进制文件,将其扔到环境变量的path下即可~
用rust打造实时天气命令行工具[1]
找到合适的API
使用该api[2]
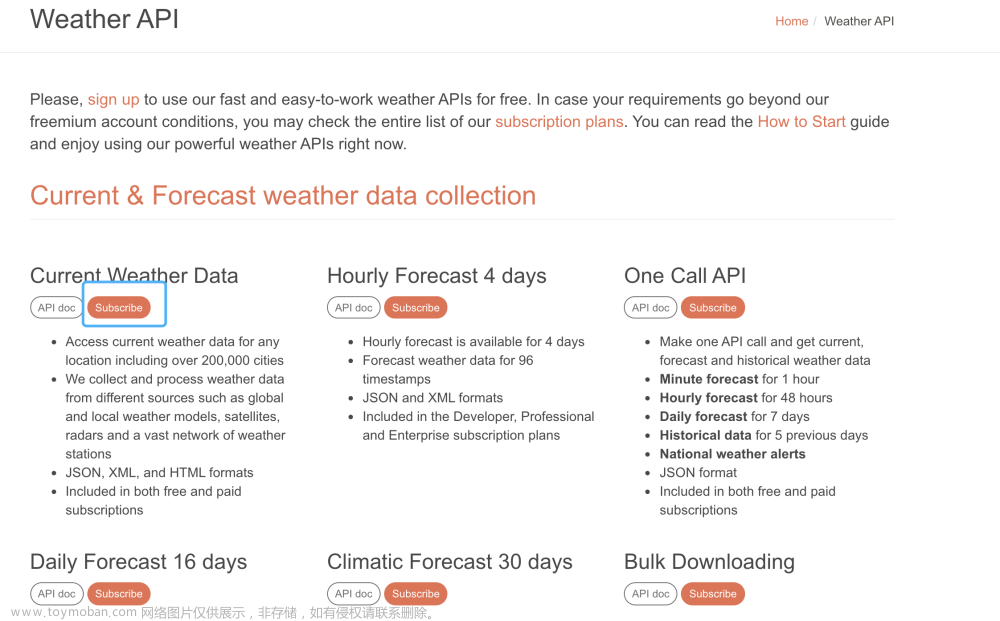
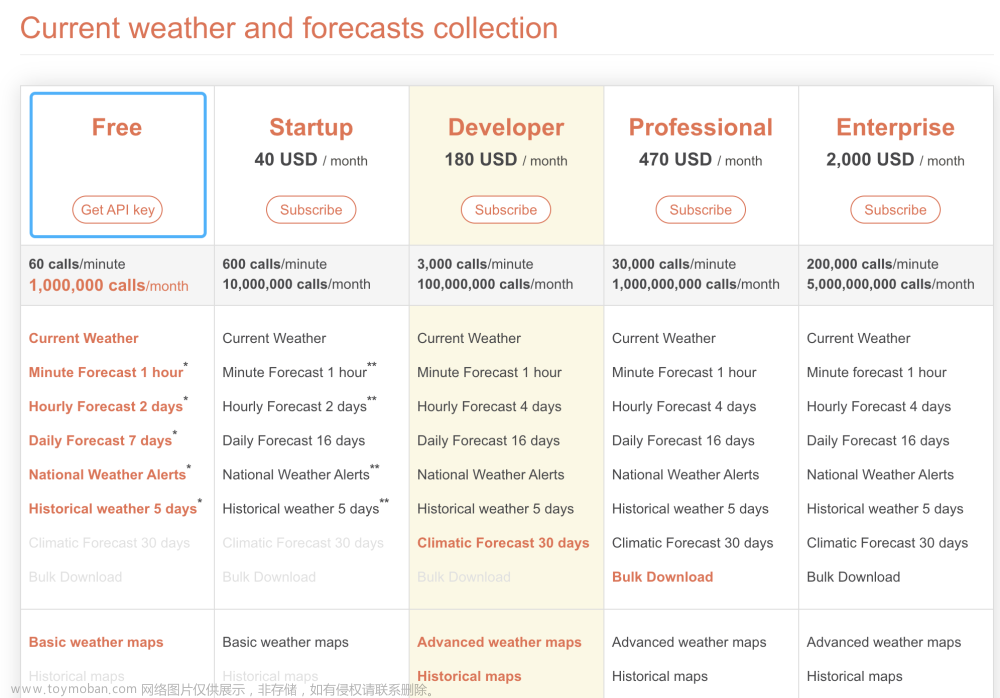
如请求 api.openweathermap.org/data/2.5/weather?q=Beijing&appid=your_key
:
{
"coord": {
"lon": 116.3972,
"lat": 39.9075
},
"weather": [{
"id": 803,
"main": "Clouds",
"description": "broken clouds",
"icon": "04d"
}],
"base": "stations",
"main": {
"temp": 293.35,
"feels_like": 292.34,
"temp_min": 291.09,
"temp_max": 294.13,
"pressure": 1026,
"humidity": 35,
"sea_level": 1026,
"grnd_level": 1020
},
"visibility": 10000,
"wind": {
"speed": 4.86,
"deg": 344,
"gust": 7.43
},
"clouds": {
"all": 73
},
"dt": 1634262993,
"sys": {
"type": 2,
"id": 2021025,
"country": "CN",
"sunrise": 1634250256,
"sunset": 1634290552
},
"timezone": 28800,
"id": 1816670,
"name": "Beijing",
"cod": 200
}
初始化项目&coding
使用cargo new rust_weather
初始化一个项目。
对于cargo.toml文件:
[package]
name = "rust_weather"
version = "0.1.0"
edition = "2018"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
structopt = "0.3.21"
exitfailure = "0.5.1"
serde = "1.0.114"
serde_json = "1.0.56"
serde_derive = "1.0.114"
reqwest = { version = "0.11", features = ["json"] }
tokio = { version = "1", features = ["full"] }
对于src/main.rs文件:
use exitfailure::ExitFailure;
use reqwest::Url;
use serde_derive::{Deserialize, Serialize};
use structopt::StructOpt;
#[derive(Serialize,Deserialize,Debug)]
struct W {
coord: Coord,
weather: Weather,
base: String,
main: Main,
}
impl W {
async fn get(city: &String) -> Result<Self, ExitFailure> {
let url = format!("https://api.openweathermap.org/data/2.5/weather?q={}&appid=40452068d845180226c3f289341974b7", city);
// 转换为url
let url = Url::parse(&*url)?;
let resp = reqwest::get(url).await?.json::<W>().await?;
Ok(resp)
}
}
#[derive(Serialize,Deserialize,Debug)]
struct Coord {
lon: f64,
lat: f64,
}
#[derive(Serialize,Deserialize,Debug)]
struct Weather {
details: Details,
}
#[derive(Serialize,Deserialize,Debug)]
struct Details {
id: i32,
main: String,
description: String,
icon: String,
}
#[derive(Serialize,Deserialize,Debug)]
struct Main {
temp: f64,
feels_like: f64,
temp_min: f64,
temp_max: f64,
pressure: i32,
humidity: i32,
}
#[derive(StructOpt)]
struct Input {
city: String
}
#[tokio::main]
async fn main() -> Result<(), ExitFailure> {
let input = Input::from_args();
//println!("{}", input.city);
let resp = W::get(&input.city).await?;
println!("{} \n 天气: {} \n 当前温度: {} \n 最高温度: {} \n 最低温度: {} \n 湿度: {}", input.city, resp.weather.details.main, resp.main.temp, resp.main.temp_max, resp.main.temp_min, resp.main.humidity);
//println!("Hello, world!");
Ok(())
}
使用cargo run Beijing
进行调试
直到能够准确输出预订结果,如下:
➜ rust_weather git:(master) ✗ cargo run Beijing
Finished dev [unoptimized + debuginfo] target(s) in 0.13s
Running `target/debug/rust_weather Beijing`
Beijing
天气: Clouds
当前温度: 293.35
最高温度: 294.13
最低温度: 291.09
湿度: 35
将二进制文件移动到系统PATH路径下
此时target/debug/rust_weather即想要的二进制文件,可将其复制到任意一个系统PATH路径下
echo $PATH
/opt/homebrew/opt/node@12/bin:/Users/fliter/.nvm/versions/node/v16.9.0/bin:/usr/local/Cellar/mysql@5.7/5.7.28/bin:/opt/homebrew/bin:/opt/homebrew/sbin:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/usr/local/go/bin:/Users/fliter/.cargo/bin:/usr/local/go/bin:/Users/fliter/go/bin:/Users/fliter/Downloads/:/bin:/usr/local/MongoDB/bin:/usr/local/Cellar/ffmpeg/4.3.1/bin:/Users/fliter/.cargo/bin
还可以重命名,如改为weather
,复制到usr/local/bin下,而后source .zshrc
在任意命令行窗口下,执行 weather Binzhou
:
Binzhou
天气: Rain
当前温度: 291.63
最高温度: 291.63
最低温度: 291.63
湿度: 67
参考自原子之音[3]
参考资料
用rust打造实时天气命令行工具: https://www.bilibili.com/video/BV1eL411b7EL
[2]该api: https://openweathermap.org/api
[3]原子之音: https://www.bilibili.com/video/BV1eL411b7EL文章来源:https://www.toymoban.com/news/detail-677853.html
本文由 mdnice 多平台发布文章来源地址https://www.toymoban.com/news/detail-677853.html
到了这里,关于使用Rust开发命令行工具的文章就介绍完了。如果您还想了解更多内容,请在右上角搜索TOY模板网以前的文章或继续浏览下面的相关文章,希望大家以后多多支持TOY模板网!