作为大一的新生,突然出现一个实训课程,让原本平静的生活变得很充实,学校要求写一个小学生四则运算考试系统的项目,包括自动出题、自动判断对错并给出得分、自动给出正确答案,同时还有倒计时,在经过几天的努力之后,我也是马马虎虎的完成了一个较为简单的考试系统
一共包含了四个类,代码如下:
下面一段代码是登陆界面的设计:
效果图:
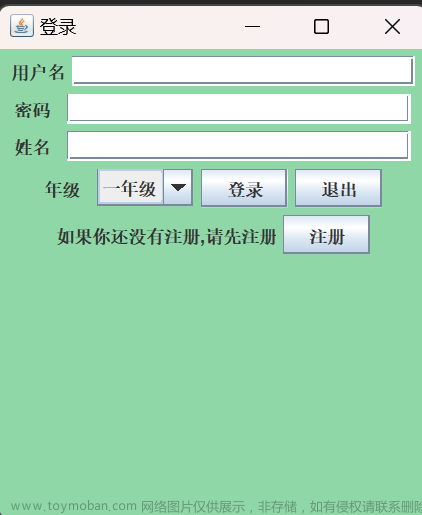
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LoginJFrame extends JFrame {
JTextField jTextField1,jTextField2;//用户名 姓名
JPasswordField jPasswordField1;//密码
JComboBox<String> nianjiJC;
public static String zhang,mi,nian;//保存账号密码
public static String zhang1,mi1,nian1;//保存账号密码
public LoginJFrame(){
init();
initzujian();
this.setVisible(true);//可见
}
//基础属性设置
private void init(){
this.setLocationRelativeTo(null);//居中
this.setSize(300,350);//大小
this.setDefaultCloseOperation(2);//关闭模式
this.setTitle("登录");
this.setLayout(new FlowLayout());
}
//界面初始化
private void initzujian(){
JLabel yonghuming = new JLabel("用户名");
JLabel mima = new JLabel("密码 ");
JLabel xingming = new JLabel("姓名 ");
jTextField1 = new JTextField(25);//用户名
jPasswordField1 = new JPasswordField(25);//密码
jTextField2 = new JTextField(25);//姓名
this.add(yonghuming);
this.add(jTextField1);
this.add(mima);
this.add(jPasswordField1);
this.add(xingming);
this.add(jTextField2);
//年级设置
JLabel nianji = new JLabel("年级 ");
String[] nj = {"一年级","二年级","三年级","四年级","五年级","六年级"};
nianjiJC = new JComboBox<>(nj);
add(nianji);
add(nianjiJC);
JButton dengluJButto = new JButton("登录");
JButton tuichuJButto = new JButton("退出");
JButton zhuceJButto = new JButton("注册");
add(dengluJButto);
add(tuichuJButto);
add(new JLabel("如果你还没有注册,请先注册"));
add(zhuceJButto);
zhuceJButto.addActionListener(new zhuceAction());//注册按钮添加事件
dengluJButto.addActionListener(new dengluAction());
tuichuJButto.addActionListener(new tuichuAction());
}
//登录按钮的事件
class dengluAction implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
boolean b1 = jTextField1.getText().equals(zhang) && jTextField2.getText().equals(mi) && nianjiJC.getSelectedItem().toString().trim().equals(nian);
boolean b2 = jTextField1.getText().equals(zhang1) && jTextField2.getText().equals(mi1) && nianjiJC.getSelectedItem().toString().trim().equals(nian1);
if (b1 || b2){
JOptionPane.showMessageDialog(null,"登陆成功");
new MainJFrame();
MainJFrame.yonghu.setText("当前用户为 :"+jTextField1.getText());
dispose();
}else if (jTextField1.getText().equals("") || jTextField2.getText().equals("")){
JOptionPane.showMessageDialog(null,"用户名或密码不能为空");
}else {
JOptionPane.showMessageDialog(null,"用户名或密码错误");
}
}
}
//退出按钮的事件
class tuichuAction implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
dispose();
}
}
//注册按钮的事件
class zhuceAction implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
dispose();
new RegisterJFrame();
}
}
public static void main(String[] args) {
new LoginJFrame();
}
}
对注册界面的设计:
效果图:
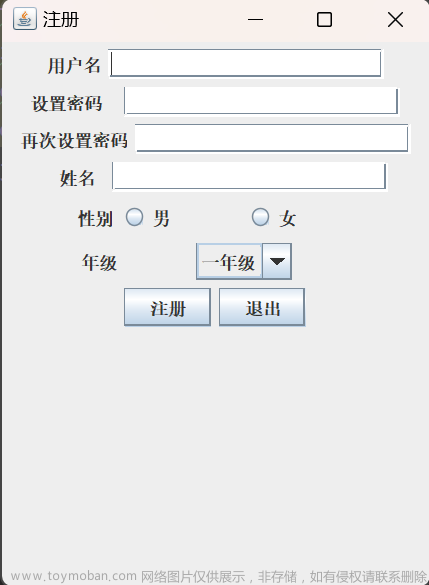
package 四则运算.考试系统界面;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class RegisterJFrame extends JFrame {
private JButton zhuceJButto,tuichuJButto;
private JLabel yonghuming,mima,mima1,xingming,xingbie;
private JTextField jTextField1,jTextFieldxingming;
private JRadioButton manJRadioButton,womanJRadioButton;
private JPasswordField jTextFieldmima,jTextFieldmima1;
private JComboBox<String> nianjiJC;
//构造方法
public RegisterJFrame(){
init();
initzujian();
initAction();
this.setVisible(true);
}
public void init(){
this.setSize(300,400);
this.setTitle("注册");
this.setLayout(new FlowLayout());
this.setLocationRelativeTo(null);
this.setDefaultCloseOperation(2);
}
public void initzujian(){
yonghuming = new JLabel("用户名");
mima = new JLabel("设置密码 ");
mima1 = new JLabel("再次设置密码");
xingming = new JLabel(" 姓名 ");
xingbie = new JLabel(" 性别");
jTextField1 = new JTextField(20);//用户名
jTextFieldxingming = new JTextField(20);//姓名
jTextFieldmima = new JPasswordField(20);//密码
jTextFieldmima1 = new JPasswordField(20);//密码1
//性别设置
manJRadioButton = new JRadioButton("男");
womanJRadioButton = new JRadioButton("女");
ButtonGroup bg = new ButtonGroup();
bg.add(manJRadioButton);
bg.add(womanJRadioButton);
this.add(yonghuming);
this.add(jTextField1);
this.add(mima);
this.add(jTextFieldmima);
this.add(mima1);
this.add(jTextFieldmima1);
this.add(xingming);
this.add(jTextFieldxingming);
add(xingbie);
add(manJRadioButton);
add(new JLabel(" "));
add(womanJRadioButton);
add(new JLabel(" "));
//年级设置
JLabel nianji = new JLabel(" 年级 ");
String[] nj = {"一年级","二年级","三年级","四年级","五年级","六年级"};
nianjiJC = new JComboBox<>(nj);
add(nianji);
add(new JLabel(" "));
add(nianjiJC);
add(new JLabel(" "));
tuichuJButto = new JButton("退出");
zhuceJButto = new JButton("注册");
add(zhuceJButto);
add(tuichuJButto);
tuichuJButto.addActionListener(new tuichuAction());
}
//事件处理的初始化
public void initAction(){
zhuceJButto.addActionListener(new zhuceAction());
}
//注册按钮的事件处理
class zhuceAction implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
if (jTextField1.getText().equals(LoginJFrame.zhang) || jTextField1.getText().equals(LoginJFrame.zhang1)){
JOptionPane.showMessageDialog(null,"该用户名已存在");
jTextField1.setText("");
}else if (jTextField1.getText().equals("") || jTextFieldmima.getText().equals("")){
JOptionPane.showMessageDialog(null,"请输入用户名和密码");
}else if (!jTextFieldmima.getText().equals(jTextFieldmima1.getText())){
JOptionPane.showMessageDialog(null,"两次输入的密码不一致");
}else if (LoginJFrame.zhang==null){
LoginJFrame.zhang = jTextField1.getText();
LoginJFrame.mi = jTextFieldmima.getText();
LoginJFrame.nian = nianjiJC.getSelectedItem().toString().trim();
JOptionPane.showMessageDialog(null,"注册成功,跳转登陆界面");
dispose();
new LoginJFrame();
}else {
LoginJFrame.zhang1 = jTextField1.getText();
LoginJFrame.mi1 = jTextFieldmima.getText();
LoginJFrame.nian1 = nianjiJC.getSelectedItem().toString().trim();
JOptionPane.showMessageDialog(null,"注册成功1,跳转登陆界面");
dispose();
new LoginJFrame();
}
}
}
//退出按钮的事件
class tuichuAction implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
dispose();
new LoginJFrame();
}
}
public static void main(String[] args) {
new RegisterJFrame();
}
}
对主界面的设计:
效果图:
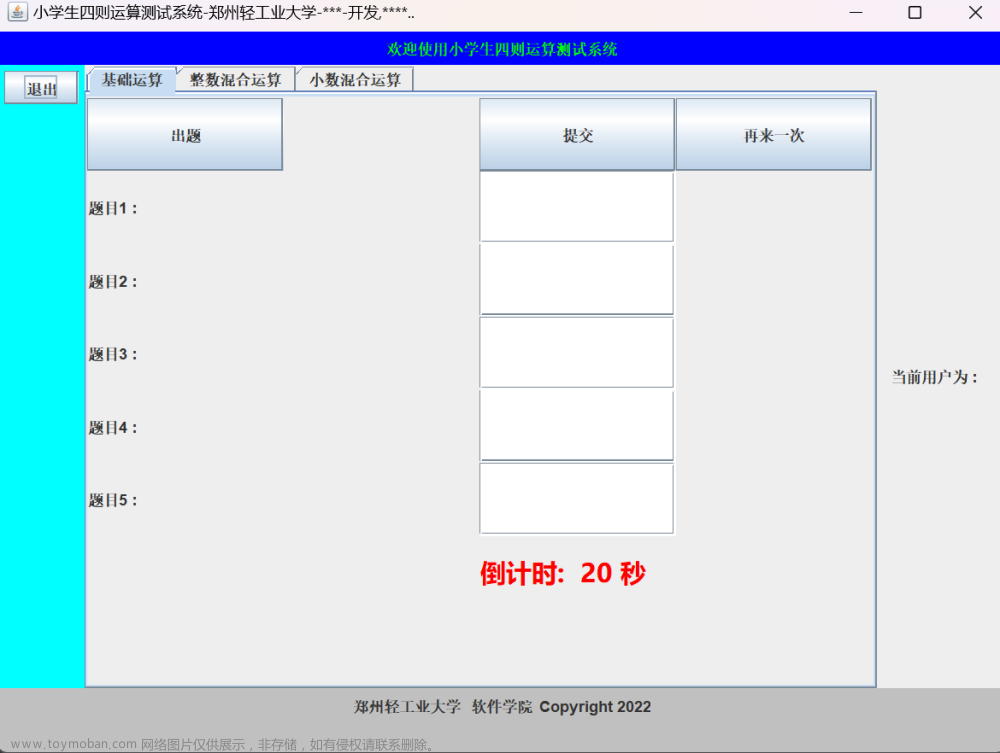
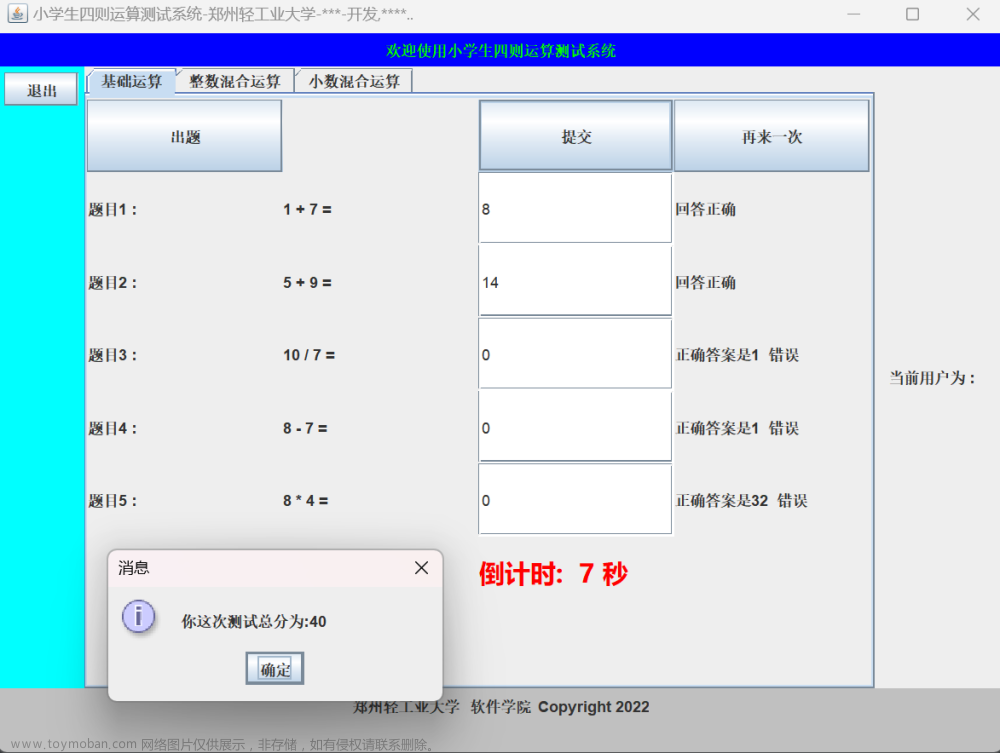
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MainJFrame extends JFrame {
//private JButton againJButton,exitJButton;//按钮属性
public static JLabel yonghu;
//构造方法,完成初始化界面
public MainJFrame() {
init();//界面基础设置
initNorth();//北侧布局
initWest();//左侧布局
initCenter();//中间界面
initEast();//东侧布局
initSouth();
this.setVisible(true);//设置可见
}
//初始化界面的方法
private void init() {
this.setSize(800, 600);//设置大小
this.setLocationRelativeTo(null);//居中
this.setDefaultCloseOperation(2);//设置关闭模式
this.setTitle("小学生四则运算测试系统-郑州轻工业大学-***-开发,****..");//设置标题
}
//左侧布局
private void initWest(){
JPanel jPanelWest = new JPanel();
jPanelWest.setBackground(Color.cyan);//背景色
//退出按钮
JButton tuichu = new JButton("退出");
jPanelWest.add(tuichu);
this.add(jPanelWest,BorderLayout.WEST);
tuichu.addActionListener(new tuichuAction());
}
//北侧布局
private void initNorth(){
//最上面的提示
JPanel jPanelNorth = new JPanel();
JLabel beixinxi = new JLabel("欢迎使用小学生四则运算测试系统");
beixinxi.setForeground(Color.green);
jPanelNorth.setBackground(Color.blue);//背景色
jPanelNorth.add(beixinxi);
this.getContentPane().add(jPanelNorth,BorderLayout.NORTH);
}
//中间布局
private void initCenter(){
JTabbedPane pcenter = new JTabbedPane();//选项卡面板
//基础运算选项卡
JPanel jichuJPanel = new jichuJPanel();//new 的对象是一个jpanel界面
jichuJPanel.setPreferredSize(new Dimension(500,500));
//整数混合运算选项卡
JPanel zhengshuhunheJPanel = new JPanel();
zhengshuhunheJPanel.setPreferredSize(new Dimension(500,500));
//小数混合运算选项卡
JPanel xiaoshuhunheJPanel = new JPanel();//整数混合运算
xiaoshuhunheJPanel.setPreferredSize(new Dimension(500,500));
pcenter.add("基础运算",jichuJPanel);
pcenter.add("整数混合运算",zhengshuhunheJPanel);
pcenter.add("小数混合运算",xiaoshuhunheJPanel);
add(pcenter,BorderLayout.CENTER);
}
//东侧布局
public void initEast(){
JPanel jPanelEast = new JPanel();
jPanelEast.setLayout(new BorderLayout());
jPanelEast.setPreferredSize(new Dimension(100,600));
yonghu = new JLabel(" 当前用户为 : ");
jPanelEast.add(yonghu,BorderLayout.CENTER);
this.add(jPanelEast,BorderLayout.EAST);
}
//南侧布局
private void initSouth(){
JPanel jPanelSouth = new JPanel();
jPanelSouth.setPreferredSize(new Dimension(100,50));
jPanelSouth.setLayout(new FlowLayout());
jPanelSouth.add(new JLabel("郑州轻工业大学 "));
jPanelSouth.add(new JLabel("软件学院"));
jPanelSouth.add(new JLabel("Copyright 2022"));
jPanelSouth.setBackground(Color.LIGHT_GRAY);
add(jPanelSouth,BorderLayout.SOUTH);
}
//退出按钮的事件
class tuichuAction implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
dispose();
new LoginJFrame();
}
}
public static void main(String[] args) {
new MainJFrame();
}
}
中间布局代码:
package 四则运算.考试系统界面;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
//基础运算界面
public class jichuJPanel extends JPanel{
public JButton chutiJButton, tijiaoJButton, zailaiJButton;
private JLabel[] JLBQuestion = new JLabel[5];
private JLabel[] test;
private JTextField[] text;
int[] score = new int[5];
private JLabel j2 = new JLabel("倒计时: 20 秒");
private JLabel[] daan = new JLabel[5];
//构造方法
public jichuJPanel() {
this.setLayout(new GridLayout(8, 4));
initJButton();//按钮初始化
initQuestion();//题目初始化
}
//按钮初始化
public void initJButton() {
chutiJButton = new JButton("出题");
tijiaoJButton = new JButton("提交");
zailaiJButton = new JButton("再来一次");
add(chutiJButton);
add(new JLabel());
add(tijiaoJButton);
add(zailaiJButton);
chutiJButton.addActionListener(new chutiAction());
zailaiJButton.addActionListener(new zaishiyiciAction());
tijiaoJButton.addActionListener(new tijiaoAction());
}
//题目初始化
public void initQuestion() {
//text是文本框 test是题目
test = new JLabel[5];
text = new JTextField[5];
for (int i = 0; i < 5; i++) {
test[i] = new JLabel("题目" + (i + 1) + " : ");
text[i] = new JTextField(15);
JLBQuestion[i] = new JLabel(" ");
add(test[i]);
add(JLBQuestion[i]);
daan[i] = new JLabel("");
add(text[i]);
add(daan[i]);
}
//对倒计时label的设置
add(new JLabel());
add(new JLabel());
j2.setForeground(Color.red);
j2.setFont(new Font("微软雅黑", Font.BOLD, 20));
add(j2);
add(new JLabel());
}
//再试一次按钮事件
class zaishiyiciAction implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
for (int i = 0; i < 5; i++) {
daan[i].setText("");
text[i].setText("");
}
i = 20;
LimitTime t = new LimitTime();
t.start();
}
}
//出题按钮的事件
class chutiAction implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
i = 20;
LimitTime t = new LimitTime();
t.start();
for (int i = 0; i < 5; i++) {
JLBQuestion[i].setText(new question().getRandom()[i]);
score[i] = question.calculation(question.getX()[i], question.getY()[i], question.getJ()[i]);
daan[i].setText("");
text[i].setText("");
}
}
}
//提交按钮的事件
class tijiaoAction implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
if (JLBQuestion[1].getText().equals(JLBQuestion[2].getText())){
JOptionPane.showMessageDialog(null,"请先出题");
}else {
defen();
i = -5;
j2.setText("答案已提交");
}
}
}
int i = 20;
//倒计时
class LimitTime extends Thread{
public void run()
{
II:
while (true){
while( i >= 0)
{
j2.setText("倒计时: "+i+" 秒");
try {
sleep(1000);
} catch (InterruptedException e) {
JFrame jf = new JFrame();
JOptionPane.showMessageDialog(jf,"出现了未知问题,请重启程序");
}
i--;
if (i<=-4){
break II;
}
}
defen();//执行提交
j2.setText("答案已自动提交");
break ;
}
}
}
//判断分数
private void defen(){
try {
int fenshu = 0;
for (int i = 0; i < 5; i++) {
// System.out.println(score[i]+" ");
if (text[i].getText().equals("")){
daan[i].setText("正确答案是"+score[i]+" 错误");
continue;
}
if (score[i] == Integer.parseInt(text[i].getText())){
daan[i].setText("回答正确");
fenshu += 20;
}else {
daan[i].setText("正确答案是"+score[i]+" 错误");
}
}
JOptionPane.showMessageDialog(null,"你这次测试总分为:"+fenshu);
}catch (Exception e2){
JOptionPane.showMessageDialog(null,"请按照正确的格式输入答案");
}
}
}
下面是对题目的设计类:
package 四则运算.考试系统界面;
public class question {
public static int[] x = new int[5];
public static int[] y = new int[5];
public static int[] j = new int[5];
//自定义方法,进行运算符的获取
public static String getOp(int k) {
String str="";
switch(k) {
case 1:str = "+";
break;
case 2:str = "-";
break;
case 3:str = "*";
break;
case 4:str = "/";
break;
}
return str;
}
//自定义方法,计算正确的答案
public static int calculation(int a, int b, int k){
int sum=0;
switch(k) {
case 1:sum=a+b;
break;
case 2:sum=a-b;
break;
case 3:sum=a*b;
break;
case 4:sum=a/b;
break;
}
return sum;
}
//获取随机数
public static String[] getRandom(){
String[] st = new String[5];
for (int i = 0; i < 5; i++) {
int a = (int)(Math.random()*10+1);
x[i] = a;
int b = (int)(Math.random()*10+1);
y[i] = b;
int k = (int)(Math.random()*4+1);//获取运算符
j[i] = k;
st[i] = a+" "+getOp(k)+" "+b+" = ";
}
return st;
}
public static int[] getX(){
return x;
}
public static int[] getY(){
return y;
}public static int[] getJ(){
return j;
}
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
System.out.println(x[i]+" "+j[i]+" "+y[i]);
}
for (int i = 0; i < 5; i++) {
System.out.println(x[i]+" "+getOp(j[i])+" "+y[i]);
}
}
}
如果要使用,只需自己创建四个类,将上面代码复制一下就可以了,注意类名要保持一致
希望大家喜欢,如果有疑问可以评论告诉我,一定会及时解答文章来源:https://www.toymoban.com/news/detail-760222.html
链接:https://pan.baidu.com/s/1lAeXTY5Pbsp7DFSevV81DQ
提取码:hmok文章来源地址https://www.toymoban.com/news/detail-760222.html
到了这里,关于小学生四则运算考试系统Java的文章就介绍完了。如果您还想了解更多内容,请在右上角搜索TOY模板网以前的文章或继续浏览下面的相关文章,希望大家以后多多支持TOY模板网!